In object-oriented programming, it is common to have separate classes for different entities or concepts. This separation of concerns allows code to be more organized and maintainable. In PHP, there are often user classes and database classes that encapsulate CRUD (create, read, update, delete) operations for users and database interactions respectively. There are several reasons why these CRUD functions are kept separate instead of combined into one class.
Separation of Concerns
Having separate user and database classes follows the separation of concerns principle in software engineering. The user class deals with user objects, authentication, sessions etc. while the database class handles interacting with the database engine like MySQL or PostgreSQL. Keeping these concerns decoupled avoids bloated classes and tight coupling.
The user class does not need to know how data is stored or persisted. Its only concern is user objects and related logic. Similarly, the database class is focused on database abstraction and querying without dealing with sessions, cookies etc. This separation enables easier testing, maintenance and extending of code over time.
Different Data and Responsibilities
The user and database classes store and interact with very different kinds of data. User classes store user objects, sessions and state while database classes work with database records organized into tables.
Their responsibilities are also very different. User classes authenticate users, maintain session state and encapsulate business logic related to users. Database classes issue queries, map records to objects, manage database connections and abstract away vendor specific syntax.
Trying to jam these two very different sets of data and responsibilities into one monolithic class would be messy and counterproductive. Splitting them leads to modular and focused classes.
Reusability
The database and user classes can be reused across different projects when coded properly. A well written database class can connect to MySQL in one project and PostgreSQL in another with minimal changes.
Also Read
Micro-moments In Mobile Commerce
How to Make a Fitness App That Inspires Users
A properly encapsulated user class can handle user registration, authentication and authorization logic across various applications. This reusability and interoperability would be diminished if the classes were tightly coupled together.
Customizability
Separating the classes also enables easier customization and swapping of implementations. For example, if an application needs to switch from MySQL to MongoDB, only the database class needs to change while the user class remains untouched.
Different types of user classes like CustomerUser or AdminUser can inherit from a base User class without affecting the database implementation. This flexibility and customizability is enabled by loose coupling between the classes.
Security
Combining user and database logic can sometimes lead to vulnerabilities and insecure practices like including credentials in code. By separating concerns, the database class can be designed with security best practices in mind without mixing in user authentication logic which has its own security considerations.
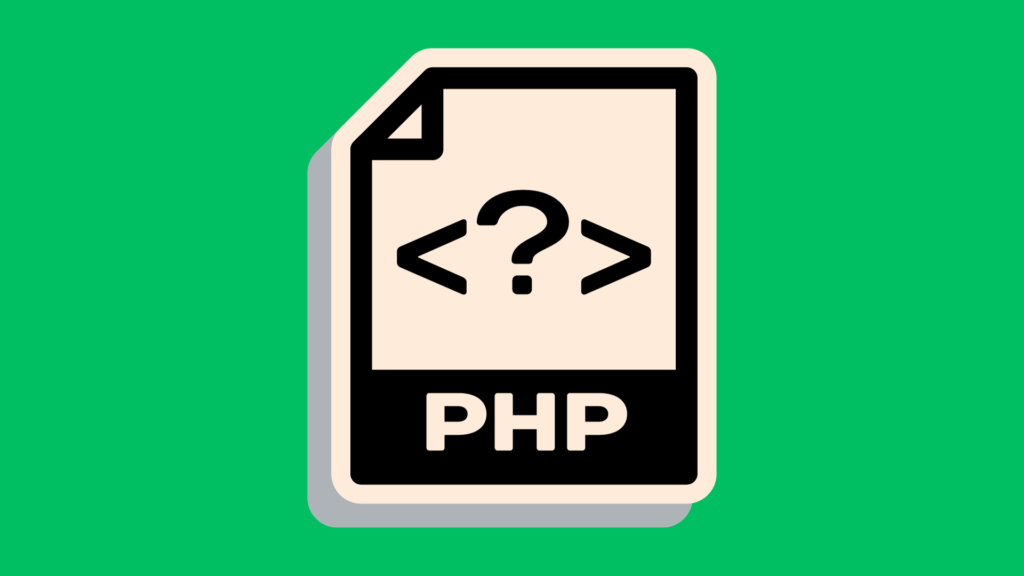
The user class can handle password hashing, salting, encryption etc. while the database class focuses on prepared statements, input validation and avoiding SQL injection. Keeping security focused code apart in this way leads to better overall security for applications.
Testability
Loosely coupled classes are much easier to test in isolation. The user class can be tested independently with mock objects instead of real database connections. This makes tests faster and more reliable.
Similarly, the database class can be tested separate from any user session logic which often involves cookies, encryption and other complications. So separation promotes easier and more robust testing.
Different Development Teams
In large projects with many developers, the user and database classes may be developed by separate teams. So it makes sense to have them separate if they are going to be designed and maintained by separate groups of developers.
Loose coupling enables different teams to focus on their classes without stepping on each other’s toes. It also allows for clear ownership by individual teams over their classes.
Modularity
Having self-contained modular classes for users and databases encourages better coding practices since the classes have clear purposes. Classes that try to do too many different things often suffer from bloat and lack of cohesion.
Separation enables the database class to handle one responsibility – database integration. And the user class is focused exclusively on user management. This modularity contributes to easier debugging, maintenance and onboarding of new developers.
Flexibility in Calling Classes
Caller classes like controllers benefit from the separation of user and database classes because they can call whichever class they need. If the controller needs to retrieve user data, it can simply call a method on the user class without having to go through database logic.
And when it needs to save data to the database, it can call the appropriate method in the database class. This separation of concerns gives a great deal of flexibility in application code.
Example Code
Here is some sample code showing how the separate user and database classes are implemented:
// User class
class User {
public function login($email, $password) {
// check password and set session
}
public function getPreferences() {
// get user prefs from session
}
}
// Database class
class Database {
public function getUser($userId) {
// query database for user
}
public function saveUser($user) {
// save user object to database
}
}
// Controller
$user = new User();
$db = new Database();
$user->login('john@example.com', 'password');
$userData = $db->getUser($user->id);
This demonstrates how the controller is able to separately call the user and database classes for user sessions and database operations. This modular separation and loose coupling enables greater flexibility and organization in application code.
Conclusion
Keeping the user and database CRUD operations in separate classes follows sound object-oriented principles and provides many benefits like modularization, flexibility, security and testability. While they could be combined, the preferred approach in PHP is to leverage loose coupling between classes by giving them focused responsibilities. This separation of concerns results in applications that are more scalable, robust and maintainable over time.